Python
Activity Goals
- Learn about the different data types and how to use them
- Learn about loops and if statements
- Learn how to write methods
Why do we use Python?
Python is a strong computational tool that is used in physics and astronomy to analyze data and run simulations. This webpage provides a basic introduction up to if statements and while loops. For more help and detailed documentation, check out Python Documentation.
Introduction
Python is a programming language commonly used in Physics and Astronomy to analyze data and run simulations. It is easy to learn, as it tends to be simple and quite descriptive. In this tutorial, we will be designing a simple number guessing game.
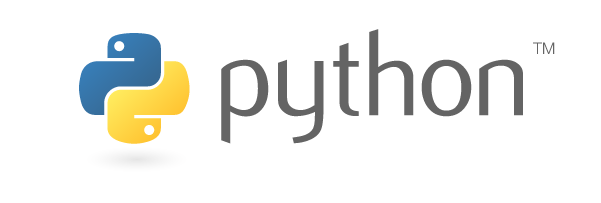
Data types
- Strings are surrounded by two quotation marks "". Strings can hold both letters or numbers, which means that
"C-3PO"
is a valid string. - Integers are whole numbers. Meaning that 16.5 is not a valid integer, but 16 is.
Note: int("34")
turns the string "34"
into an integer 34
, and int(16.5)
turns to 16
- Booleans are a very useful data type that are either
True
orFalse
. We will be talking more about them in the Logic section There are other data types that you can store which are beyond the scope of what we will be discussing in this tutorial.
Variables
- Variables are boxes in the computer’s memory that store single values. You give each variable a name and use that name to refer to the value stored in that box. Variable names can only contain letters, numbers and the underscore _.
- To create a variable, give the variable a name and assign it a value using the
=
sign. - Storing strings:
my_name = "Thanos"
means that we have made a variable calledmy_name
and given it a text value of Thanos. - Variables can store numbers and expressions that evaluate to numbers too.
For example,
body_temp = (98.6 - 32) * 5/9
evaluates the expression to37.0
, and stores it in body_temp. - One more useful value type we can store is a Boolean, either True or False.
Exercise
- Create a string variable my_name that holds your name
- Create a integer variable my_number that holds your favourite number smaller than 50
- Create a Boolean variable like_avengers that is True if you like the Avengers and False if you do not
Print and Inputs
The print()
function displays a message on the screen. print(string1, string2, ...)
- Try adding the line
print("Hello World!")
in the command Python Console print()
tells Python what it is supposed to do (in this case, print something out to the screen)."Hello World!"
is a string, a text phrase enclosed in quotes.print()
can output string values, as well as variables, as you'll see in the next section.- You can combine multiple string values into a single string with the
+
sign. Tryprint("super" + "cali" + "fragi" + "listic" + "expi" + "ali" + "docious")
- When passing in multiple values to
print()
, they are separated by spaces automatically. Tryprint("I'm", "a", "really", "long", "sentence!")
The input()
function allows you to ask the user for something and then store it as a variable.
- The input function normally takes in a string that signifies the question that you want to ask the user. For example
input("What is your name")
- Since the input function returns the value as a string, you would want to save it to a variable. For example
color = input("What is your favourite color?")
will save the value you type in into the variablecolor
. - Note that the input function saves the returned value as a string and you might have to covert that to a other types if you want to use integers for example.
Exercise 1
Write some code to ask your friend for their name, and then print the following message "Hi __Friend's name__ name! My name is __Your name__"
Exercise 2
Write some code to ask your friend for their favourite number and store it as an integer
Logical Operators
Operator | Description | Example |
---|---|---|
== | If the values of two operands are equal, then the condition becomes true. | a == b |
!= | If values of two operands are not equal, then condition becomes true. | a != b |
> | If the value of left operand is greater than the value of right operand, then condition becomes true. | a > b |
< | If the value of left operand is less than the value of right operand, then condition becomes true. | a < b |
>= | If the value of left operand is greater than or equal to the value of right operand, then condition becomes true. | a >= b |
<= | If the value of left operand is less than or equal to the value of right operand, then condition becomes true. | a <= b |
Question to Think About
x = 10<100
What do we get if we print(x)?
If Statements
In Python you can use if statements to run commands under a certain condition. For example:
age = 14
if age > 20:
print("You are old!")
2
3
If-else statements are an extended form of if statements. The else statement tells Python what should happen if the if condition is not true.
class_size = 20
apples = 0
if apples >= class_size :
print("I have enough apples for everybody!")
else:
print("I don't have enough apples!")
2
3
4
5
6
Exercise
Build on the code you have and print, "My number is bigger"/ "Same number" / "My number is smaller"
. See if your favourite number is bigger, same, or smaller than your friend's number.
Loops
for
loops are used when you want instructions to repeat a certain number of times.
For example, if we wanted to print "Hello World!" 5 times:
for i in range(5):
print("Hello World!")
2
range(5)
gives a sequence of numbers starting from 0 and up to (but not including) 5. Each iteration of the loop, it saves the number into variable i. Try replacing "Hello World!" by i and see what happens!
while
loops are used when you want instructions to repeated while a certain condition is true.
For example, if you want to print until you have 4 apples:
apples = 10
while apples > 4:
print("I have more than four apples")
apples = apples – 1
2
3
4
Question to Think About
Why did we add the last line?
Exercise
Make a number guessing game that keeps asking your friend to input a number until they guess your favourite number
Packages and Functions
We can wrap our code in what is called a Function to make it more readable (especially if we have hundreds of lines). Functions tend to have one specific purpose that sometimes can return a value to the rest of your code. An example of a function will look like this:
def function(argument_1, argument_2):
result = argument_1 + argument_2
return result
2
3
Packages are pre-written scripts that include methods that we can use for in our code. We can import them using the import keyword. For example:
import Turtle
import random
2
There are many packages that can do all sorts of things within Python. It is always a good idea to use google to check if there exists a package that does what you want.
Bonus
Play with Turtle. Use the two files, rocket.py and bonus.py (you may also find them on the Y:
drive), to complete the bonus exercise. You can find more help on Turtle Documentation